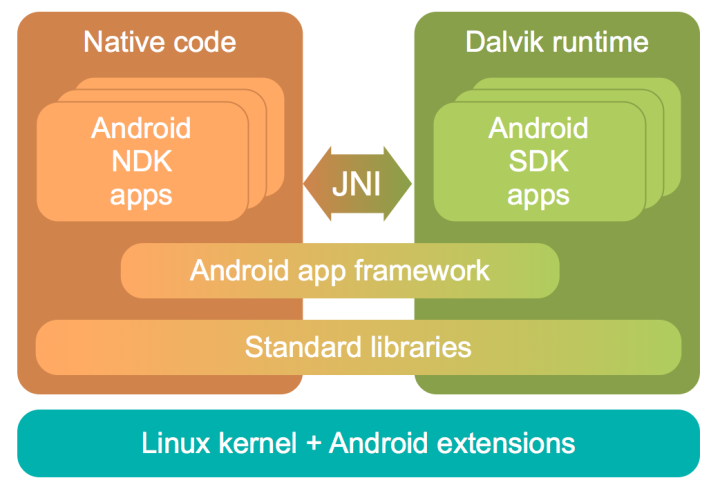
Being an illustrated run through the basics.
What happened was, for our recent South American tour I wanted an Android architecture overview graphic. I ran across, among the Android SDK documentation, a page entitled What is Android?, and it’s perfectly OK. Except for, I really disliked the picture — on purely aesthetic grounds, just not my kind of lettering and gradients and layouts — so I decided to make another one.
I thought I’d run it here and, since I’ve been spending a lot of time recently explaining What Android Is to people, I thought I’d provide my version of that as well, in narrative rather than point form.
First of all, as Dan Morrill memorably explained in On Android Compatibility, “Android is not a specification, or a distribution in the traditional Linux sense. It’s not a collection of replaceable components. Android is a chunk of software that you port to a device.”
Linux · Underneath everything is a reasonably up-to-date Linux kernel (2.6.32 in my current Nexus One running Froyo), with some power-saving extensions we cooked up; the process of trying to merge this stuff into upstream Linux has been extended and public and is by no means over. ¶
Android runs on Linux, but I’d be nervous about calling it a distro because it leaves out so much that people expect in one of those: libraries and shells and editors and GUIs and programming frameworks. It’s a pretty naked kernel, which becomes obvious the first time you find yourself using a shell on an Android device.
If it were a distro it’d be one of the higher-volume ones, shipping at 200K units a day in late 2010. But nobody counts these things, and then there are a ton of embedded flavors of Linux shipping in unremarkable pieces of consumer electronics, so there’s a refreshing absence of anyone claiming to be “the most popular Linux”. I like that.
Dalvik · The next big piece is Dalvik, comprising the VM and a whole bunch of basic runtime essentials. Its design is fairly unique, and judging by recent history, seems to be working out pretty well as a mobile-device app substrate. ¶
All the standard APIs that you use to create Android apps are defined in terms of Dalvik classes and interfaces and objects and methods. In fact, some of them are thin layers of Dalvik code over native implementations.
It’s possible, and common practice, to call back and forth between Dalvik and native code using the JNI protocol, which is a neat trick since what’s running on Dalvik isn’t anything like Java bytecodes on a Java VM.
How It’s Generated · Native code is currently produced more or less exclusively by compiling C or C++ code; but there’s no reason it has to be that way. Dalvik code is currently produced by generating Java bytecodes and translating them; but there’s no reason it has to be that way. ¶
I want to emphasize this point a little. Android apps are defined as code that runs on the platform and uses the APIs. As long as an app does these things properly, it’s really nobody’s concern how it got generated.
Special Apps · The picture is a little misleading, because some of those Dalvik-based apps are provided by Google and sometimes are seen as “part of Android”. I’m talking about the Dialer and Contacts and Calendar and Gmail and Chat and so on. Most of them are open-source and replaceable (and have been replaced by handset makers); a few are closed-source and proprietary, like Google Maps and Android Market. ¶
That Open-Source Thing · In the big picture above, most of the stuff in green is Apache-licensed. The rest is a mixture of GPL and LGPL and BSD, with some Apache in there too. This excludes some low-level device drivers and of course the majority of non-Google apps, which are closed-source. ¶
The Framework · This is the stuff that uniquely defines Android; more or less everything that Google wrote and you wouldn’t expect to find on a reasonably-configured GNU/Linux box. Its proper use is the subject of all the many pages on display at developer.android.com and of endless mailing lists, sample sites, and a growing number of books. ¶
I like it; but you already knew that.
Libraries · The word “standard” here means “generally available to programmers working in an open-source environment”. The picture isn’t comprehensive. ¶
Quite a few people, including me, have over-emphasized the role of the Harmony libraries. To start with, the Android selection excludes lots of stuff, for example AWT and Swing and OMG CORBA; all superfluous for apps using the Android framework.
Also, just counting roughly by code bulk of all the sort of stuff in this picture, the Harmony code comprises less than half the total. I don’t want to diss Harmony, they’re a wonderful project and I’m a huge fan; but it’s inaccurate to give the impression that Android is just Dalvik plus Harmony.
What’s In an App? · An Android app lives in what’s called an APK, which is simply a ZIP file, with a particular internal file layout that allows it to be run in place, without unpacking. There’s nothing magic about them, you can email them around and drop them on USB keys and extract pieces with unzip. ¶
The Android Manifest is the interface between an app and the Android system, and that’s all I’m going to say here because it’s a key piece of the puzzle and deserves lengthy discussion if any at all.
The resource bundle contains your audio and video and graphics and so on, the pieces that come with the app as opposed to being fetched over the network.
Native or Not · Most apps these days are written for Dalvik. When I say “most apps” I mean “everything that isn’t a game”; game developers typically want to code in C/C++ and that’s it. Dalvik offers a nice fast gateway to OpenGL and all the phone’s hardware, but game devs just don’t want to hear about virtual machines, so they use the Android NDK. ¶
If you’re writing code in the Java programming language you can use Eclipse and a pretty nice toolchain that makes the barrier to entry remarkably low. If you’re coding to the NDK, you’re going to be doing a lot of the build-time machinery yourself and living without some of the nice debugging and profiling candy, not to mention signing up to port your code to other CPU architectures if they run Android and have lots of users. But game developers revel in pain.
And that’s what Android is · Hope you liked the pictures. ¶
My thanks to
Dan Bornstein and
Dan Morrill for
sanity-checking this and offering several significant improvements.
[Updated:] A couple of fact-checks contributed by Brian Swetland.
Comment feed for ongoing:
From: Tachyon Feathertail (Nov 23 2010, at 12:43)
Might want to edit this:
"But game developers revel in pain.
And that’s what Android is."
[link]
From: masklinn (Nov 23 2010, at 14:14)
> But game developers revel in pain.
Or don't see the point in locking their perfectly portable backend opengl es code onto a single platform. And have real-time constraint most VMs are unable to meet.
[link]
From: Jonas (Nov 23 2010, at 14:14)
I won't believe your Nexus One is still running Froyo :P
[link]
From: Peter Keane (Nov 23 2010, at 14:23)
I've written one Android app. Not much more than "hello world" (although quite useful). It simply makes an HTTP request to a little notepad service I've created (simple PHP/MySQL) that I can edit through a web interface. I leave myself notes that I can then lookup anytime/anywhere on my phone.
I'm not a regular Eclipse/Java guy. Mostly Python & PHP. Honestly, the hassle of dealing w/ Eclipse (rather than my beloved vim) makes it highly unlikely that I'll bother writing another app (although there are lots I'd be inclined to write were it easier). Perhaps the Android NDK would be a suitable way to run CPython (?). From my POV, it would just make *much* more sense to be able to write Android apps in a simple dynamic language (I suppose Python would be top choice).
[link]
From: jojo (Nov 23 2010, at 14:38)
RE:
From: Tachyon Feathertail (Nov 23 2010, at 12:43)
Might want to edit this:
"But game developers revel in pain.
And that’s what Android is."
In your comment you are directly relating the two statements together. Anybody reading Bray's post with half a brain understands "And that’s what Android is." is a statement of closure and conclusion tying Bray's entire thesis together. As you can see in his post it is a completely different paragraph. How did you get through your English Composition classes?
[link]
From: Richard (Nov 23 2010, at 15:47)
First, Tim, nice overview - the techie side of me would maybe like it to be slightly longer and more detailed, the sane side recognises that that would miss the point...
And to Peter, two things: I've written (and published) a few Android apps, and you'll pry my vim from my cold, dead hands - absolutely nothing is stopping you writing code in the efficient programmer's editor of your choice. And you should check out the previously-mentioned-here "Scripting Layer 4 Android" (SL4A) which allows you to write python (and perl, ruby, lua, javascript, shell(!) and tcl(!!!)) scripts to run on Android. At the moment I think it's still more useful for script/utility type things, but they're working on getting to first-class-app status.
[link]
From: Jeff (Nov 23 2010, at 16:18)
I was interested to read about what Android is, but from tbray I was expecting something juicy and interesting, not a PR piece that feels like it was edited by the legal department. I dislike and distrust Oracle and I hope they loose, but in the future it would be nice to have the hacker's perspective rather than the middle manager pep talk.
[link]
From: dude (Nov 23 2010, at 19:17)
The guy complaining that Android is pain? WTF? Android is a wonderful ecosystem. And guess what? Any video game developer eeking out the cpu cycles is going to have to code for all hardware platforms, doesn't matter if it's windows, IOS, android or whatever. So your statement is just as unintelligent as it is uninformed. Good luck nimrod
[link]
From: Android User (Nov 24 2010, at 02:58)
That's a nice concise writeup.
However I feel a clarification should be made on the "open source" part. I'm not sure what to call it, but maybe "available source" is a better fit. Unlike most open source projects the development repository/trunk is walled off behind closed doors - for instance I can happily check out a copy of a Firefox 4 beta or the latest Webkit, and contribute if able, but I cannot download Gingerbread for love nor money. Similarly it is somewhat unique in that the vast majority of target hardware is locked to a particular manufacturer build (which I appreciate is largely a manufacturer issue, but the "tivoisation" really reflects on the ecosystem).
Post from my Samsung Galaxy S, stuck on 2.1 ;).
[link]
From: Thomas (Nov 24 2010, at 07:02)
Thanks for your take on it Android. I like your graphics and paired description. I think it does a much nicer job laying out what's going on. The official documentation, while more detailed, is it a little too busy to provide an nice clean overview.
[link]
From: Bernt (Nov 24 2010, at 07:18)
Questions:
* Is it possible to use the Android as a Linux development platform?
* Can I compile POSIX sources on the Android?
* For instance, can I download bash, Vim and Python tarballs to an Android device and build?
[link]
From: carlos (Nov 24 2010, at 08:31)
Heh, I initially read "OMG CORBA" as "Oh my God! CORBA" and thought that you were as surprised as I am that anyone still uses it.
[link]
From: Tushar (Nov 24 2010, at 11:45)
re: "since what’s running on Dalvik isn’t anything like Java bytecodes on a Java VM."
Can you explain more please, what does Dalvik code look like: is it just an alternative, lower-level set of bytecodes? Is it similar to LLVM or is it all compiled down to C/C++. If so, is it compiled in advance or just-in-time, etc?
[link]
From: Mike (Nov 24 2010, at 13:37)
@Android User (Nov 24 2010, at 02:58)
You're in no way stuck on 2.1. I recently acquired a Galaxy S (the Rogers Captivate) and had it running 2.2 within a few hours. Getting root access to the phone is easy, and there are a ton of custom built ROMs out there. Some of them are based on the ROMs released by the carriers, others are built from scratch AOSP.
As an aside, I took the anti-spam question (Would a bed be more likely to have sheets or leaves?) and fed it into wolfram alpha. It answered correctly.
[link]
From: Dave Walker (Nov 24 2010, at 14:18)
Concise, informative and useful Thanks for posting :-).
As has been suggested, this might form the root article of a little series, exploring some areas in more detail ;-).
[link]
From: Someone (Nov 24 2010, at 15:24)
Following "In the big picture above, most of the stuff in green is Apache-licensed." with a picture in that same green that shows a component that was reddish in the 'big picture above' technically is not incorrect, but couldn't you do better? (I think I would have gone for separate colors or hues for each license, even if it would have made the pictures uglier.)
[link]
From: BeeSauce (Nov 24 2010, at 16:22)
"But game developers revel in pain". So rewriting my entire in game in Java from C/C++ (which I chose in the first place so I could avoid having to rewrite it for each platforms) is less painful than using the NDK?
BTW, the NDK is horrible to work with, having to call back into the JVM via JNI to load and play sound effects is just pure garbage. Accessing resources in the APK via copying bytes between JVM and native code... fugly.
It's been nothing but painful developing games for Android. It's not wonder the market place is full of turds.
iOS and Palm/HP WebOS did this right. WebOS's PDK is infinitely easier to work with and of course iOS doesn't need one.
[link]
From: Dude (Nov 25 2010, at 01:57)
Which of these is responsible for sending my data to China?
SCNR ;-)
[link]
From: shan (Nov 25 2010, at 03:03)
why the extra /201x/ in the url ? faster search ?
[link]
From: God of Biscuits (Nov 25 2010, at 20:28)
"You're in no way stuck on 2.1. I recently acquired a Galaxy S (the Rogers Captivate) and had it running 2.2 within a few hours. Getting root access to the phone is easy, and there are a ton of custom built ROMs out there. Some of them are based on the ROMs released by the carriers, others are built from scratch AOSP."
Several wasted hours.
Having to root a PHONE.
finding the righ custom ROMs.
All just so you can upgrade your device's 'open source' OS to the next dot release?
Wow. Where do I sign up for this Elysium much deserved after all these years of battling the tyranny of Steven P. Jobs and his Curating Demon Minions?
[link]
From: Emmanuel Okeke (Dec 02 2010, at 00:03)
@Peter Keane as regards building android apps in a dynamic fashion, try "Titanium" from Appcelerator.
It allows you to build "native" android apps using web technologies you're already familiar with viz a viz html and javascript.
You'll love it.
twitter.com/emmanix2002
[link]
From: Jackie Duarte (Dec 30 2010, at 11:27)
Makes a lot of since, thanks for the break down
the thing I agree with most is:
"But game developers revel in pain."
I went to college for a game design major and basically the first day of programming class my teacher said basically the same thing, he also stated it was the reason for game programmers going bald though...
[link]